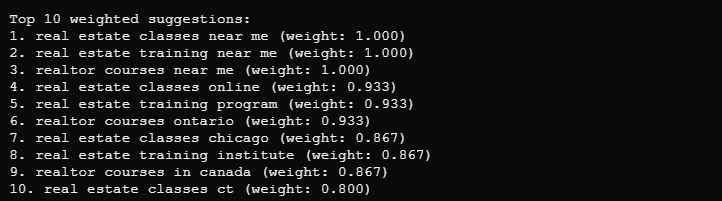
I recently developed a tool that leverages Google’s autocomplete API to analyze search patterns and user intent, particularly useful for market research and SEO analysis. Let me share how it works and what makes it interesting!
The Problem
Market researchers and SEO specialists often need to understand what people are searching for in specific regions. While Google Trends and Keyword Planners exist, I wanted a more direct approach to analyze raw search suggestions with custom weighting.
The Solution
I built a Node.js tool that:
- Fetches Google search suggestions for multiple seed keywords
- Supports location-based suggestions (geotargeting)
- Implements intelligent weighting based on suggestion positions
- Aggregates and ranks suggestions across multiple related queries
Technical Implementation
The core features include:
- Rate Limiting: Built-in protection against API rate limits using a time-delay mechanism
async waitForRateLimit() {
const currentTime = Date.now();
const timeSinceLast = currentTime - this.lastRequestTime;
if (timeSinceLast < this.minDelay) {
await new Promise(resolve =>
setTimeout(resolve, this.minDelay - timeSinceLast)
);
}
}
- Geotargeting: Support for location-based suggestions using Google’s location encoding
const params = new URLSearchParams({
client: 'chrome',
q: query,
hl: 'en',
gl: 'us'
});
- Weighted Analysis: Suggestions are weighted based on their position in results and frequency across different seed keywords
const weight = (suggestions.length - index) / suggestions.length;
Key Features
- 🌍 Geographic targeting for region-specific research
- ⚡ Efficient rate limiting to respect API constraints
- 📊 Smart weighting algorithm for result ranking
- 🔄 Cross-reference analysis across multiple keywords
Use Cases
This tool is particularly valuable for:
- Market researchers analyzing regional trends
- SEO specialists researching keyword opportunities
- Content creators looking for topic ideas
- Businesses understanding customer search patterns
Open Source
The project is available on GitHub. Feel free to check it out, contribute, or adapt it for your needs!